After the rotation fixes, one weird issue remained with Guybrush, when getting on the raft in the set
mot. When Guybrush got on the raft, he would strangely rotate in a circular motion once on the raft.
To debug the problem, I commented out the lines in the set file
mot.lua regarding guybrush getting on the raft and found that it was a call to
setrot which caused the issue to occur. In this call,
setrot was called with
TRUE as the fourth parameter, causing Guybrush to rotate over time instead of snapping to the rotation. Due to some difficulties with getting the rotation before Guybrush turned out of the retail version, I used a
textObject in the lua script before
setrot was called to display the values. Here's the retail version and ResidualVM:
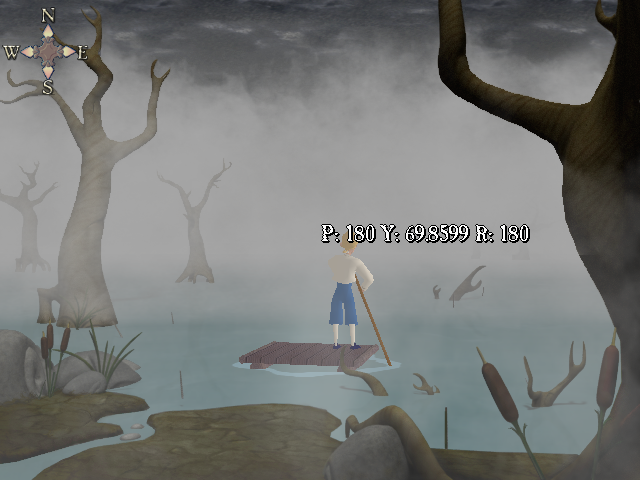 |
Guybrush's rotation before getting on the raft in the Retail Version |
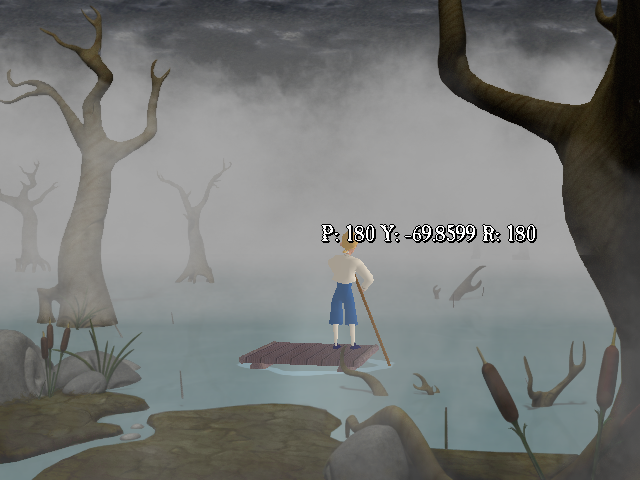 |
Guybrush's rotation before getting on the raft in ResidualVM |
As can be seen, the yaw parameter is inverted! So, is this the issue that's causing the weird rotation? I tried setting Guybrush's rotation before calling
setrot(0, 0, 0, TRUE) with
guybrush:setrot(180, 69.8599, 180) and found that the weird rotation still occurred. So, it seems there are actually two problems here:
- When rotating, Guybrush is sometimes rotated in axes that he shouldn't be when setrot is called without snapping to the new angles
- Guybrush's rotation is incorrect, with the yaw axis returning a negative value when it should be positive
To inspect the first problem, I tried setting Guybrush's rotation to 0 in each of the axes independently and inspecting the result and found that no axis was directly responsible for this rotation. I then tried setting them all to 0 with a snap and found that this looked correct (which was expected, obviously). The next experiment was to set each of the angles to a part of the rotation. I tried
(0, 69.8599, 0) first and found that the result looked right, indicating that the pitch and roll axes might be causing the problem. I then tried
(45, 69.8599, 45) and found that it also looked correct! However, at
(90, 69.8599, 90), the issue re-appeared. In fact, any value below 90 seemed to work okay, while values greater than 90 caused problems. Out of curiosity, I then tried
(-45, 69.8599, -45) and found that it also worked fine! In fact, values between -90 and 90 (non-inclusive) seemed to produce the correct result, while values outside that range produced incorrect behavior.
This pointed to a problem with the conversions between Euler angles and other rotation forms in that angles between (-90, 90) were calculated correctly, but larger angles were not. First, I checked the functions that I wrote by implementing a simple perl program to compute the rotation matrices from Euler Angles and back again. This program can be found
here and produces results demonstrating the correct equations for setting a rotation matrix from Euler Angles and retrieving the Euler Angles from the matrix:
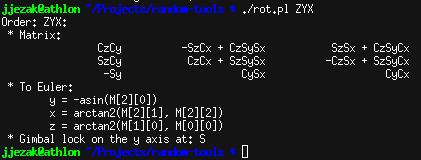 |
Output from computing the Euler -> Matrix conversion for ZYX |
Fortunately (or unfortunately for finding the bug!) the results matched and there wasn't an obvious problem, aside from the absence of checking for singularities or gimbal lock checking. However, I was having trouble keeping the X's for the axis and the X's for the Euler Angles straight, so as a part of this work, I reworked the Euler Angle nomenclature to make the naming more consistent with the usage.
With the nomenclature straightened out, I then added a fix for singularities that arise from when it's impossible to determine the correct result. For an Euler Order of ZYX, we can see that when the Y Euler Angle is
pi/2 (or
90 degrees), it will be impossible to differentiate the other two angles. We can see this by inspecting the figure above, where we can see that if the result of
Cy is 0, then the components that are used by the
arctan cannot be used to determine the result. Cy is 0 when the cosine of the Y axis angle is
pi/2 or -
pi/2. This state results in a condition called gimbal lock. To work around this issue, we can check to see if the conversion will produce gimbal lock and if so, chose a single rotation.
So, does correcting for gimbal lock fix any of our problems? We can be fairly certain that it won't just by inspection. In the scenario above, Guybrush's coordinates would not cause a singularity because the second angle (
yaw) is not +/-
90 degrees. Still, it was a necessary fix!
So, what is really going wrong here? Let's try some more experimentation! First, let's rotate Guybrush from
(0,0,0) to
(180, 0, 0) and (0,0,0) to
(0, 0, 180):
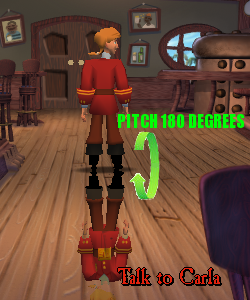 |
Pitch 180 Degrees - (0,0,0) to (180, 0, 0) |
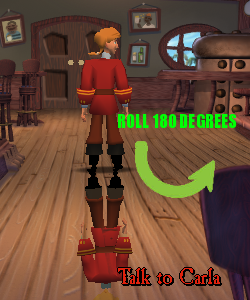 |
Roll 180 Degrees - (0,0,0) to (0, 0, 180) |
|
|
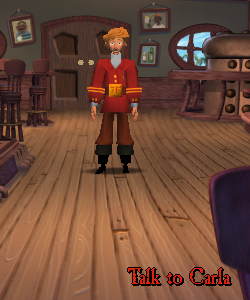 |
Rotation of (0,0,0) to (180, 0, 180) |
We can see that the sum of these rotations is going to result in Guybrush facing 180 degrees opposite his position on the yaw axis from the
(0,0,0) rotation. So, we can see that a rotation from (180, 0, 180) to (0, 0, 0) should only produce rotation in the yaw axis instead of rotating through the pitch and roll axes as well.
What causes this extra rotation? In ResidualVM, the turn methods of the Actor use Euler Angles to compute the rotation by making the angles equal. If instead, a Quaternion is used for rotation, this problem should be resolved. Now that the problem has been identified, in the next post, I'll address the issue.